When creating a new order, the order date should default to the current date.
There are three types of business rules that can be used for this purpose. Choose any one of the suggested implementations below.
SQL Business Rule
Switch back to the Project Designer. In the Project Explorer, open the Controllers tab. Right-click on Orders / Business Rules node, and press New Business Rule option.
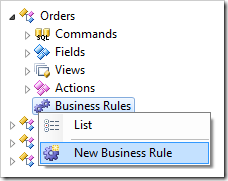
Specify the following values:
Property | New Value |
Type | SQL |
Command Name | New |
Phase | Execute |
Script | set @OrderDate = getdate()
|
Press OK to save the business rule. Press Browse in the top left corner, and wait for the page to load. Navigate to Order Form, and create a new order. Select a customer and employee. You will see that the current date has been inserted into the Order Date field.
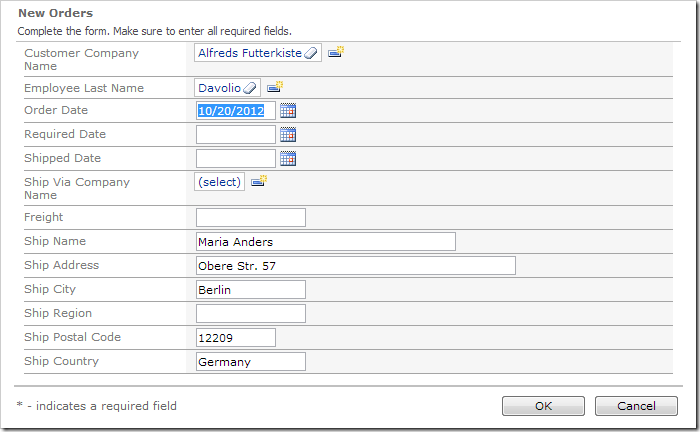
Code Business Rule
C# or Visual Basic may be used as an alternative to SQL.
Switch back to the Project Designer.
If you have implemented the business rule above, then delete it. In the Project Explorer, right-click on Orders / Business Rules / New (SQL / Execute) – r100 node, and press Delete.
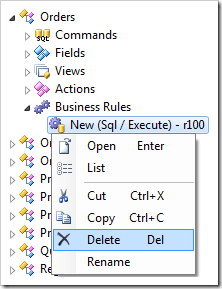
Create a “code” business rule with the following properties:
Property |
New Value |
Type |
C# / Visual Basic |
Command Name |
New |
Phase |
Execute |
Press OK to save.
Code business rules cannot be directly edited using the Project Designer, and do not exist until the project has been regenerated.
On the toolbar, press Browse to regenerate the project.
When complete, right-click on Orders / Business Rules / New (Code / Execute) – r100 node, and press Edit Rule in Visual Studio.
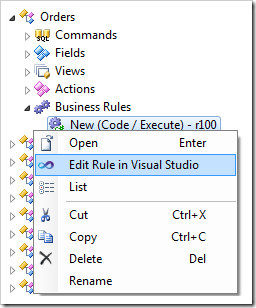
The rule file will be opened in Visual Studio. The entire class definition and parameters of the business rule method are already defined. Replace the body of the rule with the UpdateFieldValue method:
C#:
using System;
using MyCompany.Data;
namespace MyCompany.Rules
{
public partial class OrdersBusinessRules : MyCompany.Data.BusinessRules
{
[Rule("r100")]
public void r100Implementation(
int? orderID,
string customerID,
string customerCompanyName,
int? employeeID,
string employeeLastName,
DateTime? orderDate,
DateTime? requiredDate,
DateTime? shippedDate,
int? shipVia,
string shipViaCompanyName,
decimal? freight,
string shipName,
string shipAddress,
string shipCity,
string shipRegion,
string shipPostalCode,
string shipCountry)
{
UpdateFieldValue("OrderDate", DateTime.Now);
}
}
}
Visual Basic:
Imports MyCompany.Data
Imports System
Namespace MyCompany.Rules
Partial Public Class OrdersBusinessRules
Inherits MyCompany.Data.BusinessRules
<Rule("r100")> _
Public Sub r100Implementation( _
ByVal orderID As Nullable(Of Integer),
ByVal customerID As String,
ByVal customerCompanyName As String,
ByVal employeeID As Nullable(Of Integer),
ByVal employeeLastName As String,
ByVal orderDate As Nullable(Of DateTime),
ByVal requiredDate As Nullable(Of DateTime),
ByVal shippedDate As Nullable(Of DateTime),
ByVal shipVia As Nullable(Of Integer),
ByVal shipViaCompanyName As String,
ByVal freight As Nullable(Of Decimal),
ByVal shipName As String,
ByVal shipAddress As String,
ByVal shipCity As String,
ByVal shipRegion As String,
ByVal shipPostalCode As String,
ByVal shipCountry As String)
UpdateFieldValue("OrderDate", DateTime.Now)
End Sub
End Class
End Namespace
Save the file. If you create a new order in the web application, the Order Date will be populated with the current date.
JavaScript Business Rule
Business rules can also be written in JavaScript if default values can be calculated entirely on the client.
If you created one of the above business rule implementations, delete it now.
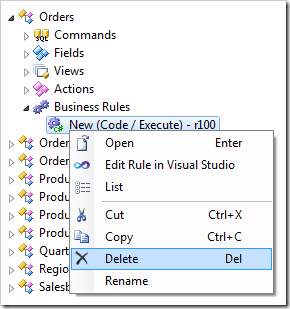
Create another business rule with this configuration:
Property |
New Value |
Type |
JavaScript |
Command Name |
New |
Phase |
After |
Script |
[OrderDate] = new Date();
|
Note that the business rule must execute after the command “New”, because the record is not instantiated on the client until after the command is executed by the application framework on the server.
Save the file. The business rule will work as shown in the illustration at the top.