Many action require additional information that must be requested from the user. The Implementing a Custom Action tutorial in Getting Started series shows an example of such action.
The built-in Report action allows implementing custom report output created on the server. Developers can use external software or write code to produce the output.
Let’s consider requesting custom parameters and processing them when producing the output. The example assumes that there is an external URL that can accept ReportHeader. The action handler will redirect user to that URL.
The action handler will obtain several parameters from the following sources
- Confirmation data controller
- Selected row of the data view
- URL in the address bar of the browser
Confirmation Controller Configuration
Make sure that Reporting is enabled for your Northwind sample project.
Start the Project Designer. In the Project Explorer, switch to the Controllers tab and press New Controller icon on the toolbar.
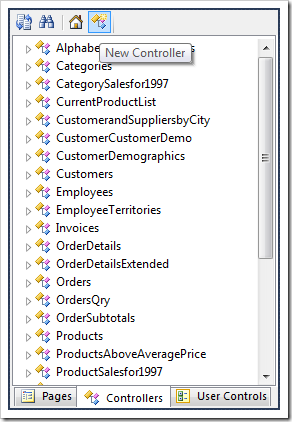
Assign a name to the controller.
Property | Value |
Name | ReportProperties |
Press OK to save the controller. Right-click on ReportProperties / Fields node, and press New Field.
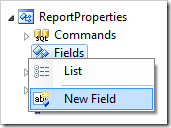
Give this field the following settings.
Property | Value |
Name | ReportHeader |
Type | String |
Length | 40 |
Allow null values | True |
Label | Report Header |
Press OK to save the field.
Adding Custom Report Action
Right-click on Orders / Actions / ag3 – New action group node, and press New Action.
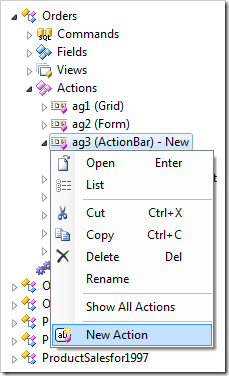
Give this action the following settings.
Property | Value |
Command Name | Report |
Command Argument | _blank |
Confirmation | _controller=ReportProperties _title=Configure properties for the report. _width=500 |
Press OK to save the action.
Handling the Action
Create a controller business rule to handle the report action.
Right-click on Orders / Business Rules node.
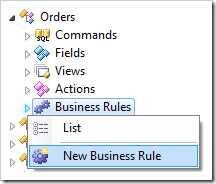
Assign the following properties.
Property | Value |
Type | C# / Visual Basic |
Command Name | Report |
Command Argument | _blank |
Phase | Execute |
Press OK to save. On the toolbar, press Generate to create the business rule file.
When finished, right-click on Orders / Business Rules / Report, _blank (Code / Execute) - r100 controller node, and press Edit Rule in Visual Studio.
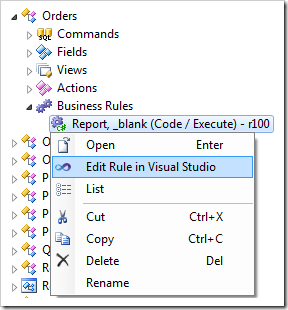
Visual Studio will load the project and display the source code file of the business rule. Replace the code with the following:
C#:
using System;
using MyCompany.Data;
namespace MyCompany.Rules
{
public partial class OrdersBusinessRules : MyCompany.Data.BusinessRules
{
/// <summary>
/// This method will execute in any view for an action
/// with a command name that matches "Report" and argument that matches "_blank".
/// </summary>
[Rule("r100")]
public void r100Implementation(
int? orderID,
string customerID,
string customerCompanyName,
int? employeeID,
string employeeLastName,
DateTime? orderDate,
DateTime? requiredDate,
DateTime? shippedDate,
int? shipVia,
string shipViaCompanyName,
decimal? freight,
string shipName,
string shipAddress,
string shipCity,
string shipRegion,
string shipPostalCode,
string shipCountry,
// custom arguments
string parameters_ReportHeader, // comes from confirmation
string xyz // comes from URL
)
{
// This is the placeholder for method implementation.
Result.NavigateUrl =
String.Format("~/Pages/OrdersReport.aspx?ReportHeader={0}&OrderID={1}&xyz={2}",
parameters_ReportHeader, orderID, xyz);
}
}
}
Visual Basic:
Imports MyCompany.Data
Imports System
Namespace MyCompany.Rules
Partial Public Class OrdersBusinessRules
Inherits MyCompany.Data.BusinessRules
''' <summary>
''' This method will execute in any view for an action
''' with a command name that matches "Report" and argument that matches "_blank".
''' </summary>
<Rule("r100")>
Public Sub r100Implementation( _
ByVal orderID As Nullable(Of Integer),
ByVal customerID As String,
ByVal customerCompanyName As String,
ByVal employeeID As Nullable(Of Integer),
ByVal employeeLastName As String,
ByVal orderDate As Nullable(Of DateTime),
ByVal requiredDate As Nullable(Of DateTime),
ByVal shippedDate As Nullable(Of DateTime),
ByVal shipVia As Nullable(Of Integer),
ByVal shipViaCompanyName As String,
ByVal freight As Nullable(Of Decimal),
ByVal shipName As String,
ByVal shipAddress As String,
ByVal shipCity As String,
ByVal shipRegion As String,
ByVal shipPostalCode As String,
ByVal shipCountry As String,
ByVal parameters_ReportHeader As String,
ByVal xyz As String
)
'This is the placeholder for method implementation.
Result.NavigateUrl =
String.Format("~/Pages/OrdersReport.aspx?ReportHeader={0}&orderId={1}&xyz={2}",
parameters_ReportHeader, orderID, xyz)
End Sub
End Class
End Namespace
Save the file.
Testing the Report Action
Switch back to the Project Designer. On the toolbar, press Browse.
Navigate to the Orders page. Append the URL parameter “?xyz=Value” to the current page.
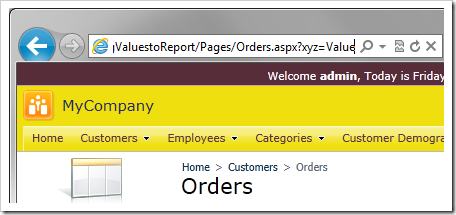
Press Enter to navigate to the URL. On the list of orders, highlight an order and activate the Report action on the action bar.
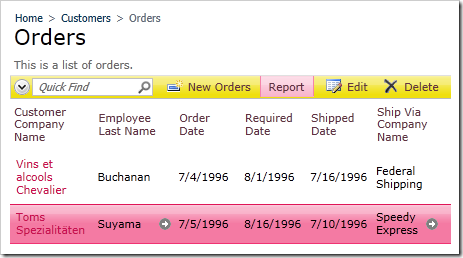
The confirmation controller will open. Enter a Report Header and press OK.
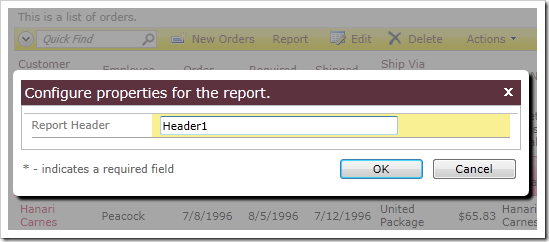
The next page will display a server error – no page was created to handle the request. However, if you look at the URL, you will see that the values of fields specified in the business rule have been passed.
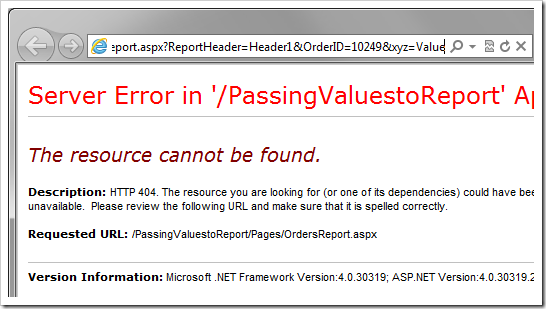
The values available for passing include already present URL parameters (xyz), a field value from the selected row (orderId), and the value specified by the user in a confirmation controller (reportHeader).
If no row was selected, the value from the first row in the grid will be passed.
If you do have a real application behind the URL, then one can expect the correct output to be produced.